Python is one of the most beginner-friendly programming languages, it is very famous for its simplicity and readability. The robust data types of Python are the foundation of its adaptability and simplicity. In this tutorial, we will discuss Python data types.
What Are Data Types?
In programming, a data type defines the type of data that a variable can hold. It specifies the range of values that the variable can take and the operations that can be performed on it.
Python Data Types
Python, as a dynamically typed language, provides a wide range of built-in data types that can be classified into several categories:
1. Numeric Types
There are 3 types of numeric data types in Python.
- int
- float
- complex
int: Represents integers
The int data type in Python represents whole numbers without any decimal point. It can be used to perform arithmetic operations like addition, subtraction, multiplication, and division. Integers can be positive, negative, or zero. They have unlimited precision and can be created using the int() constructor or by directly assigning a value
count = 50
age = int(26) # using int constructor
float: Represents floating-point numbers
The float data type in Python represents decimal numbers. It is used to store real numbers, such as 3.14 or -0.5. Floating-point numbers can be manipulated using mathematical operations.
temperature = 29.5
Complex: Represents complex numbers
A complex data type in Python represents complex numbers, which consist of a real part and an imaginary part. Complex numbers are written in the form a + bj, where a is the real part and b is the imaginary part. Example:
complex_num = 3 + 2j
2. Boolean Type
The Python boolean data type is used to represent two values: True and False. It is commonly used in conditional statements and logical operations. Booleans are case-sensitive and must be written as True or False, with the first letter capitalized.
is_recording = False
3. Text Sequence Type
Textual data is represented by the str data type in Python.
Str: Represents strings of characters
The str data type in Python is used to represent a sequence of characters. It is immutable, it means its value cannot be changed once created. Strings can be created using single quotes double quotes or triple quotes. Various methods are available to manipulate and operate on string objects.
For example, a string created using single quotes
blog_name = 'Nolowiz'
A string created using double quotes
blog_name = "Nolowiz"
For example, a string created using triple quotes
blog_description = '''Technology tutorials,news,tips '''
4. Sequence Types
Sequence data types in Python are used to store multiple values in an ordered manner. The main sequence types are str, lists, tuples, and ranges. Different sequence data types are :
- list
- tuple
- range
List: Represents ordered, mutable sequences
The Python list data type allows you to store and manipulate a collection of items. You can add, remove, or modify elements using various methods such as append(), pop(), and insert(). Lists are mutable, meaning they can be modified after creation.
odd_numbers = [1,3,5,7]
Tuple: Represents ordered, immutable sequences
A tuple in Python is an immutable sequence data type that can store multiple elements. It is created using parentheses and elements are separated by commas. Tuples are commonly used to group related data together and can be accessed using indexing or unpacking.
numbers = (10,20,30)
Range: Represents ordered, mutable sequences
The range data type in Python is used to generate a sequence of numbers. It is commonly used in “for” loops to iterate over a specific range of values. The range function takes start, stop, and step parameters to define the sequence.
numbers = range(5)
for i in range(5):
print(i)
This will print the numbers 0 to 4. You can read more about the range function here.
5. Mapping Type
The Python dict data type is a collection of key-value pairs, where each key is unique. It allows you to store and retrieve values based on their associated keys. Dicts are mutable and can be modified using various methods like adding, updating, or deleting key-value pairs.
To create a dictionary in Python, use curly braces {} and separate key-value pairs with colons. For example: my_dict = {'key1': 'value1', 'key2': 'value2'}
. You can also use the dict() constructor or add key-value pairs using the square brackets.
For example :
person = {"name": "Alice", "age": 30}
6. Set Types
Different types of set data types are :
- set
- frozen set
Set: Represents an unordered collection of unique elements
The Python set data type allows you to store a collection of unique elements. It is mutable and unordered. You can add, remove, and perform operations like union, intersection, and difference on sets.
To create a set in Python, you can use the set() function or place items inside curly braces {}. For example:
my_set = {1, 2, 3}
another_set = set([1,2,3])
Frozenset: Represents an immutable set
The frozenset data type in Python is an immutable version of the set data type. It is created using the frozenset() function and cannot be modified once created. It is useful for storing and manipulating unique elements, just like the set data type.
my_set = {1, 2, 3}
my_frozenset = frozen
set(my_set)print(my_frozenset)
7. Binary Sequence Types
Python supports different binary sequence types.
- bytes
- bytearray
- memoryview
Bytes: Represents a sequence of bytes
The bytes data type in Python represents a sequence of byte values. It is immutable and used to store binary data like images or network packets.
To create a bytes data type in Python, you can use the bytes() constructor or by prefixing a string with b. Here’s an example:
b_data = bytes([65, 66, 67])
print(b_data)
Output
b'ABC'
In the above example, the value of numbers([65,66,67)] must be between in range (0 to 255). So b_data = bytes([65, 66, 712]) is invalid because 712 is outside the specified range.
b_data = b'Hello'
print(b_data)
Output
b'Hello'
Bytearray: Represents a mutable sequence of bytes
The bytearray data type in Python is a mutable sequence that represents a collection of integers ranging from 0 to 255. It allows for efficient manipulation of binary data, such as encoding and decoding.
To create a bytearray in Python, use the bytearray() constructor and pass in the desired length or an iterable object containing integers. Here’s an example:
# Creating a bytearray with length 5
my_bytearray = bytearray(5)
# Creating a bytearray from a list of integers
my_bytearray = bytearray([1, 2, 3, 4, 5])
Memoryview: Allows you to access the underlying memory of an object
The memoryview data type in Python allows direct access to the internal memory of an object, such as a bytearray. It provides a way to view and manipulate the data without creating a new copy.
To create a memoryview object in Python, you can use the memoryview() function. It takes an object that supports the buffer protocol as an argument. Here’s an example of using memoryview with a bytearray:
data = bytearray(b'Hello, world!')
view = memoryview(data)
print(view[7:12])
Please note that memoryview requires objects that support the buffer protocol.
8. None Type
The Python None data type represents the absence of a value. It is often used to indicate that a variable or function does not have a specific value. For example, x = None assigns the variable x to the value None.
Type Conversion
Python allows you to convert between different data types, either implicitly or explicitly. Implicit conversion occurs automatically when Python converts one type to another to perform an operation, while explicit conversion is done explicitly by the programmer using functions like int()
, str()
, or float()
.
# Implicit conversion
result = 5 + 2.0 # int + float = float
print(result) # Output: 7.0
# Explicit conversion
number = "42"
number = int(number) # Convert string to int
print(number) # Output: 42
Python data types can be listed as a table :
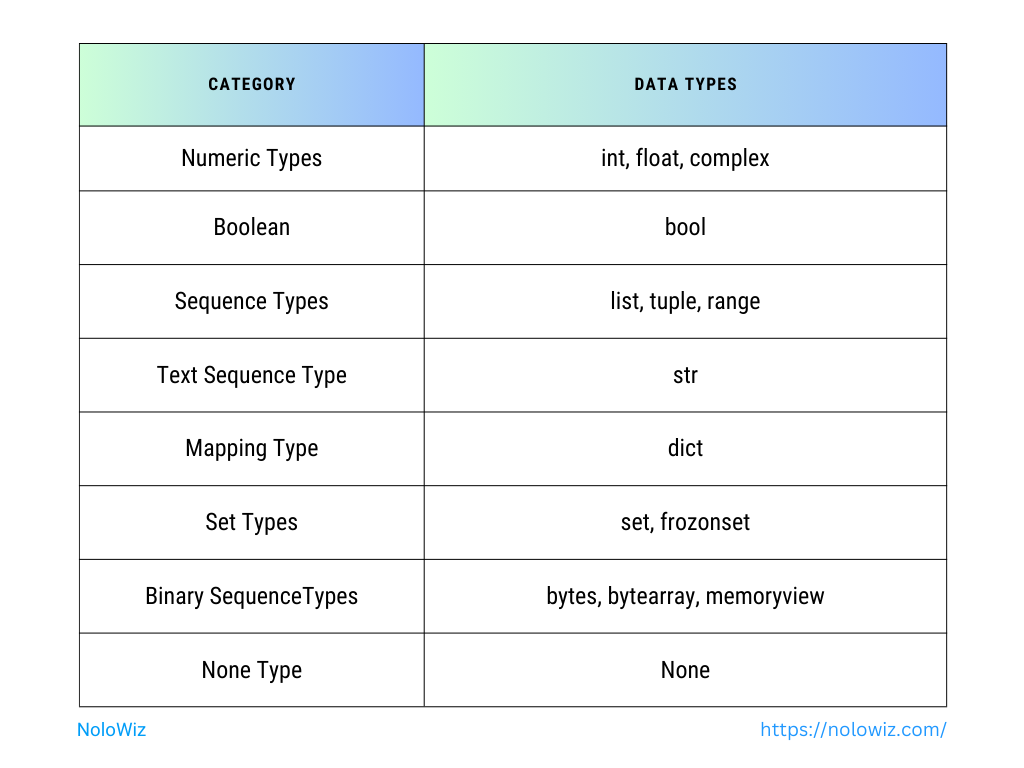
Conclusion
Data types are the foundation of Python programming, enabling you to work with various kinds of data effectively.