Python offers different flow control statements. The While loop is one of the flow control statements used in Python. In this tutorial, we will learn about while loops in Python with examples.
The while loop
The while loop is used for the repeated execution of code as long as the condition is true.
Syntax of while loop:
while condition:
# If condition is true then this code block will run
# It possible to write break or continue statement in loop body
else:
# Body of else
We can see there is an “else” clause in the while loop. However, It is optional if we give an else statement it will be executed (if the condition is false) and the loop terminates.
Inside the while loop, we can use a break or continue statements.
- break – terminates the while loop without executing the else case.
- continue – continue statement is executed and skips the rest of the while loop code and goes back to the testing condition.
Flowchart of while loop as shown below. The dotted lines are optional code, like the body of the “else” clause, break, etc
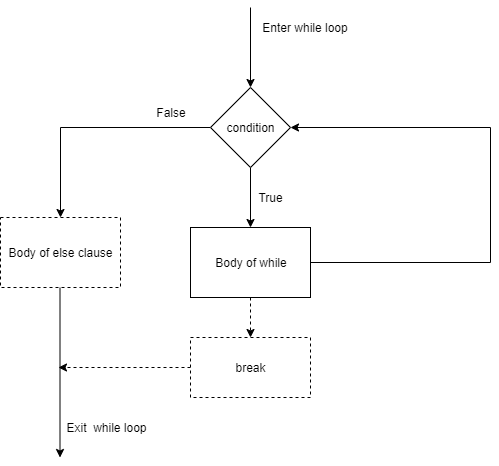
Examples
Let’s look into some examples of while in Python.
While loop example without else
While loop to print “hello” 10 times.
count = 0
while count < 10:
# body of while loop
print("Hello")
count += 1
Output
Hello
Hello
Hello
Hello
Hello
Hello
Hello
Hello
Hello
Hello
While…else example
Printing 5 odd numbers, we can see that when the condition becomes false “else” code will be executed.
count = 0
odd_number = 1
while count < 5:
# body of while loop
print(odd_number)
odd_number += 2
count += 1
else:
print("Finished")
Output
1
3
5
7
9
Finished
While loop with a break statement
In this example, while loop body has a break statement. Break loop if the number is 5.
count = 0
odd_number = 1
while count < 5:
# body of while loop
print(odd_number)
odd_number += 2
if odd_number == 5: #exit loop if number is 5
break
count += 1
else:
print("Finished")
Output
1
3
We can see that the break statement causes the while loop to exit without executing the else clause.
While loop with a continue statement
Here continue statement is present in the while loop body. It skips the code below the continue statement.
count = 0
odd_number = 1
while count < 5:
# body of while loop
odd_number += 2
if odd_number <= 9: #Print only if number > 9
continue
print(odd_number)
count += 1
else:
print("Finished")
Output
11
13
15
17
19
Finished
Conclusion
Hope this tutorial helps you to understand while loops in python. Read about 10 easter eggs in Python.